mirror of
https://github.com/MillironX/nf-core_modules.git
synced 2024-09-21 15:52:05 +00:00
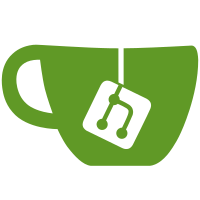
* Update LAST to version 1238. * Update functions.nf to the latest devel version. * Update test MD5sums after updating software version. * Make portable on MacOS * Allow input alignments to be uncompressed. While the strategy in this family of modules is to make all inputs and outputs compressed, this change might be useful to some users. As of LAST 2138, `last/split` does not allow its input to be compressed. * Search for .des file, that is guaranteed to be unique. Some LAST indexes have more than one .bck file and it makes the name detection crash. In this commit, I also standardise how the names are detected. * Use value input channel and optional output channels to handle formats. As discussed on Slack, it is preferred to use a value input channel instead of sneaking options through `params.args2` or `params.format` as we did. Likewise, optional output channels with clearly labeled format are preferred to 'catch-all' wildcards.
68 lines
2.5 KiB
Text
68 lines
2.5 KiB
Text
//
|
|
// Utility functions used in nf-core DSL2 module files
|
|
//
|
|
|
|
//
|
|
// Extract name of software tool from process name using $task.process
|
|
//
|
|
def getSoftwareName(task_process) {
|
|
return task_process.tokenize(':')[-1].tokenize('_')[0].toLowerCase()
|
|
}
|
|
|
|
//
|
|
// Function to initialise default values and to generate a Groovy Map of available options for nf-core modules
|
|
//
|
|
def initOptions(Map args) {
|
|
def Map options = [:]
|
|
options.args = args.args ?: ''
|
|
options.args2 = args.args2 ?: ''
|
|
options.args3 = args.args3 ?: ''
|
|
options.publish_by_meta = args.publish_by_meta ?: []
|
|
options.publish_dir = args.publish_dir ?: ''
|
|
options.publish_files = args.publish_files
|
|
options.suffix = args.suffix ?: ''
|
|
return options
|
|
}
|
|
|
|
//
|
|
// Tidy up and join elements of a list to return a path string
|
|
//
|
|
def getPathFromList(path_list) {
|
|
def paths = path_list.findAll { item -> !item?.trim().isEmpty() } // Remove empty entries
|
|
paths = paths.collect { it.trim().replaceAll("^[/]+|[/]+\$", "") } // Trim whitespace and trailing slashes
|
|
return paths.join('/')
|
|
}
|
|
|
|
//
|
|
// Function to save/publish module results
|
|
//
|
|
def saveFiles(Map args) {
|
|
if (!args.filename.endsWith('.version.txt')) {
|
|
def ioptions = initOptions(args.options)
|
|
def path_list = [ ioptions.publish_dir ?: args.publish_dir ]
|
|
if (ioptions.publish_by_meta) {
|
|
def key_list = ioptions.publish_by_meta instanceof List ? ioptions.publish_by_meta : args.publish_by_meta
|
|
for (key in key_list) {
|
|
if (args.meta && key instanceof String) {
|
|
def path = key
|
|
if (args.meta.containsKey(key)) {
|
|
path = args.meta[key] instanceof Boolean ? "${key}_${args.meta[key]}".toString() : args.meta[key]
|
|
}
|
|
path = path instanceof String ? path : ''
|
|
path_list.add(path)
|
|
}
|
|
}
|
|
}
|
|
if (ioptions.publish_files instanceof Map) {
|
|
for (ext in ioptions.publish_files) {
|
|
if (args.filename.endsWith(ext.key)) {
|
|
def ext_list = path_list.collect()
|
|
ext_list.add(ext.value)
|
|
return "${getPathFromList(ext_list)}/$args.filename"
|
|
}
|
|
}
|
|
} else if (ioptions.publish_files == null) {
|
|
return "${getPathFromList(path_list)}/$args.filename"
|
|
}
|
|
}
|
|
}
|